How Parallel Streams work?
Parallel Streams use the Fork/Join Framework under the hood to process the stream parallelly.
The Fork/Join Framework got introduced as part of Java 7 and it is an implementation of the ExecutorService interface that helps you take advantage of multiple processors.
The Fork/Join Framework distributes tasks to worker threads in a thread pool.
So the Parallel Stream implementation uses the Fork/Join to create multiple threads.
How many threads can be created?
The number of threads that can be created when we call some terminal operation together with the parallel() method or Collection.parallelStream() depends on the number of processors that are available in the machine.
Usually, it is the number of threads == the available number of processors.
How can we check how many available processors we have?
Open your IDE and write the following code to check how many available processors your machine has:
class Test { public static void main(String[] args) { System.out.println(Runtime.getRuntime().availableProcessors()); } }
My output is 8 because the CPU of my machine has 4 cores, and each core has 2 threads, so 4 * 2 = 8.
Have a look at one simple example:
IntStream.rangeClosed(1, 100000) .parallel() .sum();
Here, the sum() method is a terminal operation that starts the execution of a stream. Then, the parallel() method will split the data into multiple parts and process them concurrently.
Sequential/Regular Stream vs Parallel Stream execution flow:
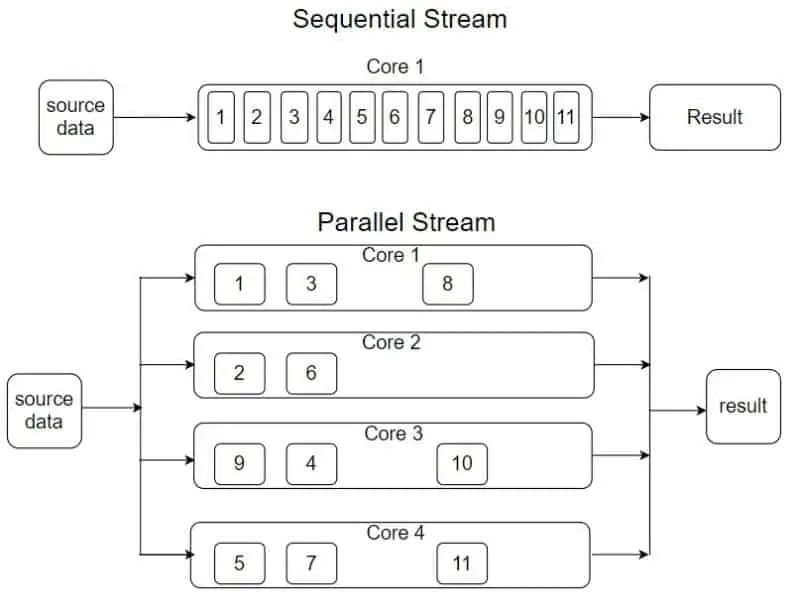
That was all about exploring the internal working of the Parallel Streams in Java. Proceed to the next lesson.
Happy Learning!