Object cloning in Java means making a copy of an object. By cloning an object we get a new object with the same data and fields as the original one.
There are two types of object cloning in Java:
- Shallow cloning
- Deep cloning
Let’s explore each of them.
Shallow cloning
When we do a shallow copy of an object, all references to other objects that the original object contains will be copied. And both objects will have references to the same objects.
If we change the value of one object, it will be reflected in another.
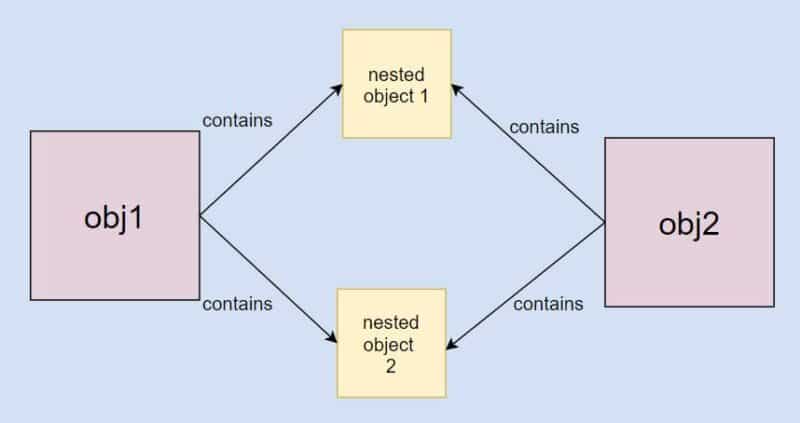
We use the clone() method from the Object class for shallow cloning.
Syntax:
protected Object clone() throws CloneNotSupportedException
The class whose object we want to clone must implement the Cloneable interface. Otherwise, we will get CloneNotSupportedException.
Object cloning using the clone() method
Let’s create one class that overrides the clone() method from the Object class and implements the Cloneable interface. The class will also have one reference to another object.
class ClassA implements Cloneable { private int someNum; private ClassC nestedObjectReference; @Override public Object clone() throws CloneNotSupportedException { return super.clone(); } // getter and setter methods } class ClassC { private String classData; public ClassC(String classData) { this.classData = classData; } // getter and setter methods }
Here, the ClassA has a reference to the ClassC.
Now, let’s make a copy of the ClassA:
public class Test { public static void main(String[] args) throws CloneNotSupportedException { ClassA obj1 = new ClassA(); obj1.setSomeNum(5); obj1.setNestedObjectReference(new ClassC("one")); ClassA obj2 = (ClassA) obj1.clone(); System.out.println("Object 1..."); System.out.println(obj1.getSomeNum()); System.out.println(obj1.getNestedObjectReference().getClassData()); System.out.println(); System.out.println("Object 2..."); System.out.println(obj2.getSomeNum()); System.out.println(obj2.getNestedObjectReference().getClassData()); } }
public class Test { public static void main(String[] args) throws CloneNotSupportedException { ClassA obj1 = new ClassA(); obj1.setSomeNum(5); obj1.setNestedObjectReference(new ClassC("one")); ClassA obj2 = (ClassA) obj1.clone(); obj2.getNestedObjectReference().setClassData("two"); System.out.println(obj1.getNestedObjectReference().getClassData()); System.out.println(obj2.getNestedObjectReference().getClassData()); } }
Advantages of shallow copy
- It is a very efficient and reliable way of object cloning widely used in practice.
- We don’t need to write our logic. We override the clone() method from the Object class and implement the Cloneable interface.
This was one way how to clone an object in Java, and in most cases, the shallow copy will be good for your purposes, but if you need something more complex, then use deep cloning.
Now, let’s explore deep cloning in the next lesson → Java deep copy