The switch statement in Java is used in cases where we have many choices to test, and in such cases, we would have to write nested if-else statements.
There are also switch expressions if you are interested to learn more.
The switch statement is better than multi-level if-else statements because it is much clearer and, in most cases, faster. That is mainly because the switch expression is evaluated only once.
The flow looks like this:
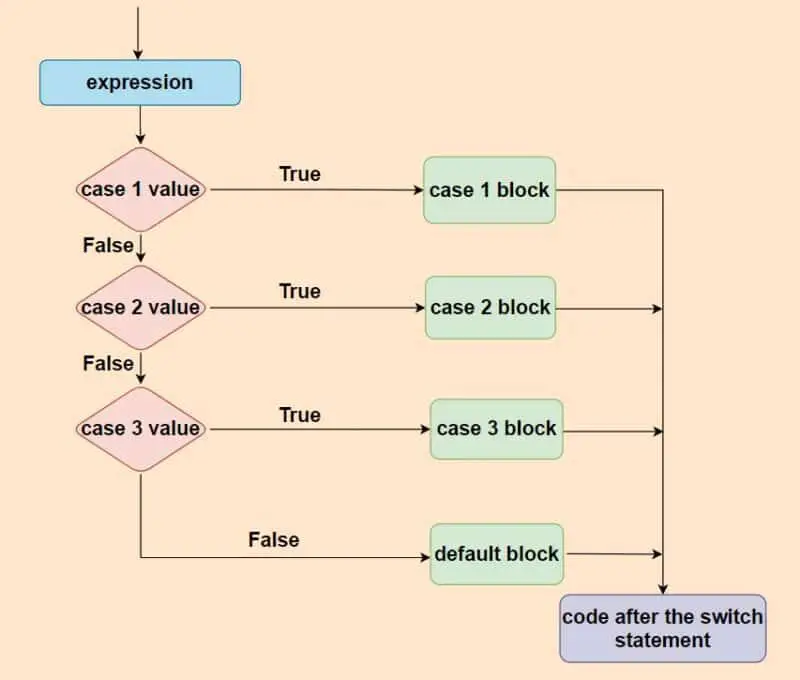
The syntax of the switch statement
switch (expression) { case constant: // switch block 1... break; case constant: // switch block 2... break; default: // default switch block ... ; }
The switch works with the byte, short, char, and int primitive data types. It also works with enumerated types (Enum), the String class, and a few wrapper classes: Character, Byte, Short, and Integer.
How does the switch statement work?
See the following example:
class Test { public static void main(String[] args) { String color = "blue"; switch (color) { case "white": // if it is white, execute this block of code System.out.println("The color is white."); break; case "yellow": // if it is yellow, execute this block of code System.out.println("The color is yellow."); break; case "blue": // if it is blue, execute this block of code System.out.println("The color is blue."); break; case "green": // if it is green, execute this block of code System.out.println("The color is green."); break; default: // None of the above is true? Then run the default block System.out.println("Unrecognized color!"); } } }
- The expression is evaluated once and compared with the values of each case label,
- If there is a match, the corresponding code gets executed,
- If there is no match, the code under default: gets executed.
Let’s see another example:
class Test { public static void main(String[] args) { int number = 10; int newNumber; switch (number) { case 5: // if the number is equal to 5, execute this block of code newNumber = number; break; case 20: // if the number is equal 20, execute this block of code newNumber = number; break; default: // None of the above is true? Then run the default block newNumber = 0; } // outside the switch statement System.out.println(newNumber); } }
break statement in Java switch cases
As you probably noticed, we used a break statement in each case block. This is to ensure that code execution stops after the matching case’s block code have been executed.
If the break statement is not used, all the cases after the matching case get executed:
class Test { public static void main(String[] args) { String color = "yellow"; switch (color) { case "white": System.out.println("The color is white."); case "yellow": System.out.println("The color is yellow."); case "blue": System.out.println("The color is blue."); case "green": System.out.println("The color is green."); default: System.out.println("Unrecognized color!"); } } }
class Test { public static void main(String[] args) { String color = "red"; switch (color) { case "white": System.out.println("The color is white."); break; default: System.out.println("Unrecognized color!"); break; case "yellow": System.out.println("The color is yellow."); break; case "blue": System.out.println("The color is blue."); break; } } }
Example:
String str = "alegrucoding"; final int lengthOfTen = 10; int lengthOfTwelve = 12; switch (str.length()) { case lengthOfTen: // OK System.out.println("The length is 10"); break; case lengthOfTwelve: // DOESN'T COMPILE: "Constant expression required" System.out.println("The length is 12"); default: // ... }
That’s it!