Exception handling is an essential part of any robust software application, and Java is no exception. In Java, an exception is an event that interrupts the normal flow of a program and can occur at runtime. It could be due to various reasons, such as incorrect input, lack of resources, or programming errors.
Exception handling helps a program handle such unexpected events gracefully and recover from them without crashing. In this tutorial, we will explore the basics of exception handling in Java, including the types of exceptions, the mechanism for handling them, and best practices for writing exception-handling code.
By the end of this tutorial, you will have a solid understanding of how to write exception-safe Java programs.
What are Exceptions in Java?
Exceptions in Java are events that occur during the execution of a program and disrupt the normal flow of instructions. When an exception occurs, the program execution is interrupted, and an error message is displayed to the user. Exceptions can occur due to various reasons, such as:
- User input errors: These include any errors caused by the user, such as invalid input data, wrong input format, or incorrect user actions.
- Device errors: Hardware or network devices can fail, causing exceptions. For example, a printer may be turned off or out of paper, or a web page may be temporarily unavailable.
- Physical limitations: Resources such as disks, memory, or CPU time can be exhausted, causing exceptions.
- Code errors: A method may not perform correctly, causing exceptions. Examples include computing an invalid array index, trying to find a non-existent entry in a hash table, or trying to pop an empty stack.
In Java, we can handle exceptions using the exception handling mechanism. By handling exceptions, we can prevent the program from crashing and display a meaningful error message to the user.
In Java, we have two types of throwable objects: errors and exceptions.
- Errors are serious issues that typically indicate a problem beyond the control of the application. Examples of errors include out-of-memory errors, stack overflow errors, and virtual machine crashes. When an error occurs, it is recommended to let the program crash instead of trying to handle it. The reason is that errors usually indicate a severe problem that cannot be recovered from.
- Exceptions, on the other hand, are issues that can be handled within the application. Exceptions can occur due to various reasons, such as user input errors, device errors, or code errors. When an exception occurs, it disrupts the normal flow of the program, but it can be caught and handled using the exception handling mechanism in Java.
It is up to the developers to protect the code as much as possible so that in the event of an exception, they can either stop the program and write a meaningful message that helps identify the cause of the error or write code that will prevent the program from crashing. Proper exception handling can prevent unexpected errors from causing damage to the application or data and help provide a better user experience.
Throwable Class Hierarchy in Java
In Java, all exception and error types are subclasses of the Throwable
class. There are two main branches of the Throwable
class hierarchy:
Exception
: This class is used for exceptional conditions that user programs should catch and handle. Exceptions are typically caused by external factors, such as user input errors, device errors, or network issues, and can be recovered from by the program.Error
: This class is used for problems that arise beyond the control of the user or the developer. Errors are typically caused by internal factors, such as out-of-memory errors or hardware failures, and cannot be easily recovered from. Therefore, it is recommended to let the program terminate when an error occurs rather than trying to handle it.
It is important to note that both Exception
and Error
are subclasses of the Throwable
class, which means that they can be caught and handled in the same way. However, the approach to handling exceptions and errors should be different. We should catch and handle exceptions to ensure that the program continues to run smoothly, while we should allow errors to terminate the program gracefully.
In summary, understanding the differences between Exception
and Error
classes is essential in designing robust Java programs. By handling exceptions correctly, we can make our programs more reliable and user-friendly while allowing errors to terminate the program can help prevent unpredictable behaviour and data corruption.
The following image summarizes the Throwable
class hierarchy in Java, showing the two main branches of Exception
and Error
, along with examples of the types of exceptions and errors that can occur.
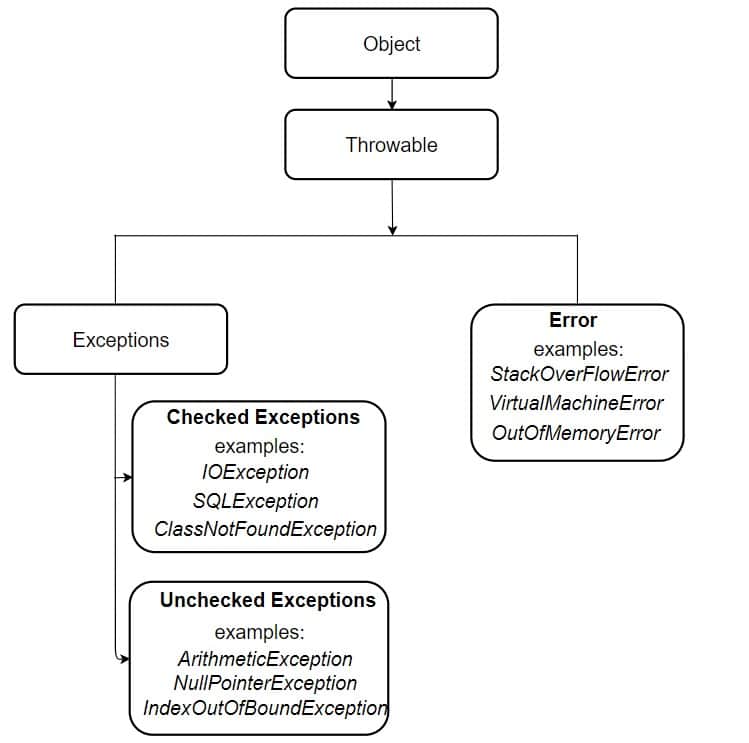
Types of Exceptions in Java
Exception handling in Java is essential for gracefully handling errors and unexpected situations that can occur during program execution. Java provides several types of exceptions that can be used to handle different types of errors. These exceptions can be broadly categorized into three types:
- Checked Exceptions
- Must be declared in the method signature using the “throws” keyword or handled within the method using a try-catch block
- Checked at compile-time
- Examples include:
- IOException: thrown when there is an error reading or writing a file
- SQLException: thrown when there is an error accessing a database
- ClassNotFoundException: thrown when a class is not found at runtime
- Unchecked Exceptions
- Do not need to be declared or caught explicitly in the code
- Also known as runtime exceptions
- Occur during program execution and are not checked by the compiler
- Examples include:
- NullPointerException: thrown when a null reference is accessed
- ArrayIndexOutOfBoundsException: thrown when an array index is out of bounds
- ClassCastException: thrown when an object is cast to an incompatible class
- Errors
- Similar to exceptions but caused by more serious problems such as system failures or resource exhaustion
- Generally not recoverable and not recommended to be caught or handled in code
- Examples include:
- OutOfMemoryError: thrown when the JVM runs out of memory
- StackOverflowError: thrown when the call stack exceeds its maximum size
- VirtualMachineError: thrown when there is an error in the JVM itself
In summary, understanding the different types of exceptions and how to handle them properly is critical for developing reliable and robust Java applications.
Exception Handling Mechanism
Exception handling is an essential aspect of Java programming as it helps handle runtime errors that might occur during program execution. In this section, we’ll explore the various mechanisms provided by Java to handle exceptions.
The try-catch block is the primary mechanism used to handle exceptions in Java. The try block encloses the code that might throw an exception, and the catch block catches the exception and executes code to handle the exception. The catch block specifies the type of exception it catches, and if an exception of that type is thrown, the code in the catch block is executed.
For example, consider the following code:
try { // code that might throw an exception } catch (ExceptionType e) { // code to handle the exception }
In this example, if an exception of type ExceptionType
is thrown within the try block, the code in the catch block is executed to handle the exception.
Multiple catch blocks can be used to handle different types of exceptions that might be thrown by the try block. In this case, each catch block specifies the type of exception it catches. When an exception is thrown, Java checks each catch block in sequence until it finds a catch block that can handle the exception.
For example:
try { // code that might throw an exception } catch (IOException e) { // code to handle IOException } catch (SQLException e) { // code to handle SQLException }
In this example, if an IOException
is thrown within the try block, the first catch block is executed to handle it. If a SQLException
is thrown, the second catch block is executed instead.
Nested try-catch blocks can also be used to handle exceptions in more complex scenarios. In this case, a try block is enclosed within another try block, and each try block has its catch block to handle exceptions.
The finally
block can be used to execute code that should always run, regardless of whether an exception was thrown or not. The code in the finally
block is executed after the try-and-catch blocks have finished executing.
For example:
try { // code that might throw an exception } catch (Exception e) { // code to handle the exception } finally { // code that always runs }
In this example, the code in the finally
block is executed after either the try block or catch block, depending on whether an exception was thrown or not.
That concludes our overview of the exception-handling mechanism in Java. Remember that proper exception handling is critical to ensure that your programs can handle unexpected errors gracefully and continue to function correctly.
Throwing Exceptions
In Java, you can throw an exception when a particular condition occurs that disrupts the normal flow of your program. The throw
keyword is used to explicitly throw an exception in your code.
The throw
Keyword
The throw
keyword is used to throw an exception explicitly. You can use it with any of the built-in or custom exception classes. For example, let’s say you have a method that checks whether a given number is even or odd. If the number is odd, you want to throw an exception. Here’s how you can do it using the throw
keyword:
public void checkEven(int num) throws OddNumberException { if(num % 2 != 0) { throw new OddNumberException("Number is odd."); } }
Here, OddNumberException
is a custom exception class that you can define in your code. If the number passed to the checkEven
method is odd, an instance of OddNumberException
will be thrown with the message “Number is odd.”
Creating Custom Exceptions
In addition to the built-in exceptions in Java, you can also create your own custom exception classes. This can be useful if you want to handle specific exceptions in a different way than the built-in exceptions.
To create a custom exception, you need to extend the Exception
class or one of its subclasses. Here’s an example of a custom exception class:
public class OddNumberException extends Exception { public OddNumberException(String message) { super(message); } }
This OddNumberException
class extends the Exception
class and has a constructor that takes a message as a parameter. You can use this class to throw an exception when a number is odd, as shown in the previous example.
Checked vs Unchecked Exceptions
In Java, there are two types of exceptions: checked and unchecked. Checked exceptions are those that the compiler forces you to handle in some way. Unchecked exceptions are those that the compiler does not require you to handle explicitly.
When you create a custom exception, you can choose whether it should be checked or unchecked. If you extend the Exception
class or one of its subclasses, your exception will be checked. If you extend the RuntimeException
class or one of its subclasses, your exception will be unchecked.
It’s generally recommended to use checked exceptions for exceptional conditions that can be handled gracefully by the caller of your code. Use unchecked exceptions for programming errors that the caller cannot reasonably recover from.
In summary, throwing exceptions is a powerful feature in Java that allows you to handle exceptional conditions gracefully in your code. You can use the throw
keyword to throw built-in or custom exceptions, and you can create your own custom exception classes to handle specific exceptions in a different way than the built-in exceptions.
Best Practices for Exception Handling
-
Avoiding empty catch blocks: An empty catch block is one that catches an exception but doesn’t do anything with it. This is a bad practice because it makes it difficult to diagnose and fix the underlying problem. It’s important to always include some code that handles or logs the exception, even if it’s just printing out a message.
-
Catching specific exceptions: It’s good practice to catch specific exceptions rather than catching all exceptions with a generic “catch-all” block. This allows you to handle different types of exceptions differently, and can make your code more robust and easier to debug.
-
Logging exceptions: Logging exceptions is an important practice because it helps you diagnose problems with your code. When an exception is thrown, log a message that includes the exception details, such as the type of exception, the message, and the stack trace. This can help you pinpoint the source of the problem and fix it more quickly.
-
Proper use of finally block: The finally block is always executed, regardless of whether an exception is thrown or not. This makes it a good place to put code that needs to be executed regardless of the outcome of the try block. For example, if you’re working with I/O streams, you should always close the streams in the finally block to ensure that they are properly released.
-
Rethrowing exceptions: Sometimes it’s appropriate to catch an exception, do some processing, and then rethrow the exception so that it can be handled further up the call stack. This can be useful for propagating the exception to a higher-level handler that has more information about how to handle the exception.
By following these best practices, you can ensure that your exception handling code is robust, maintainable, and easy to debug.
Conclusion
In summary, we have discussed the fundamentals of exception handling in Java, including the different types of exceptions, how to handle them, and best practices for writing exception-handling code. By using these techniques, you can improve the robustness and maintainability of your Java programs. With this knowledge, you can start writing better code that handles errors and exceptions more effectively.
Frequently asked questions
- Can you catch exceptions in Java lambdas?
Yes, you can catch exceptions in Java lambdas using try-catch blocks. However, you should be careful when doing this, as it can affect the readability and maintainability of your code. Additionally, catching exceptions in a lambda may also affect its functional purity. - How do you rethrow an exception in Java, and when is it appropriate to do so?
In Java, you can rethrow an exception by catching it and then using thethrow
keyword to throw it again. This is often appropriate when you want to propagate an exception up the call stack to a higher-level handler that has more information about how to handle it. For example, if you are writing a library, you might catch an exception in a low-level method and rethrow it so that the client code that uses the library can handle it appropriately. By rethrowing an exception, you can ensure that it is handled in the appropriate context and that the root cause of the problem is addressed. - Can you catch multiple exceptions in a single catch block in Java?
Yes, you can catch multiple exceptions in a single catch block in Java by using the pipe (|) operator to separate the exception types. This technique is useful when you want to handle different exceptions in the same way or when you want to avoid duplicating code in multiple catch blocks. However, be careful not to catch too many exceptions in a single catch block, as it can make your code less readable and harder to debug. - How do you throw a custom exception in Java?
To throw a custom exception in Java, you need to define a new class that extends theException
class or one of its subclasses. Then, you can use thethrow
keyword to throw an instance of your custom exception class.